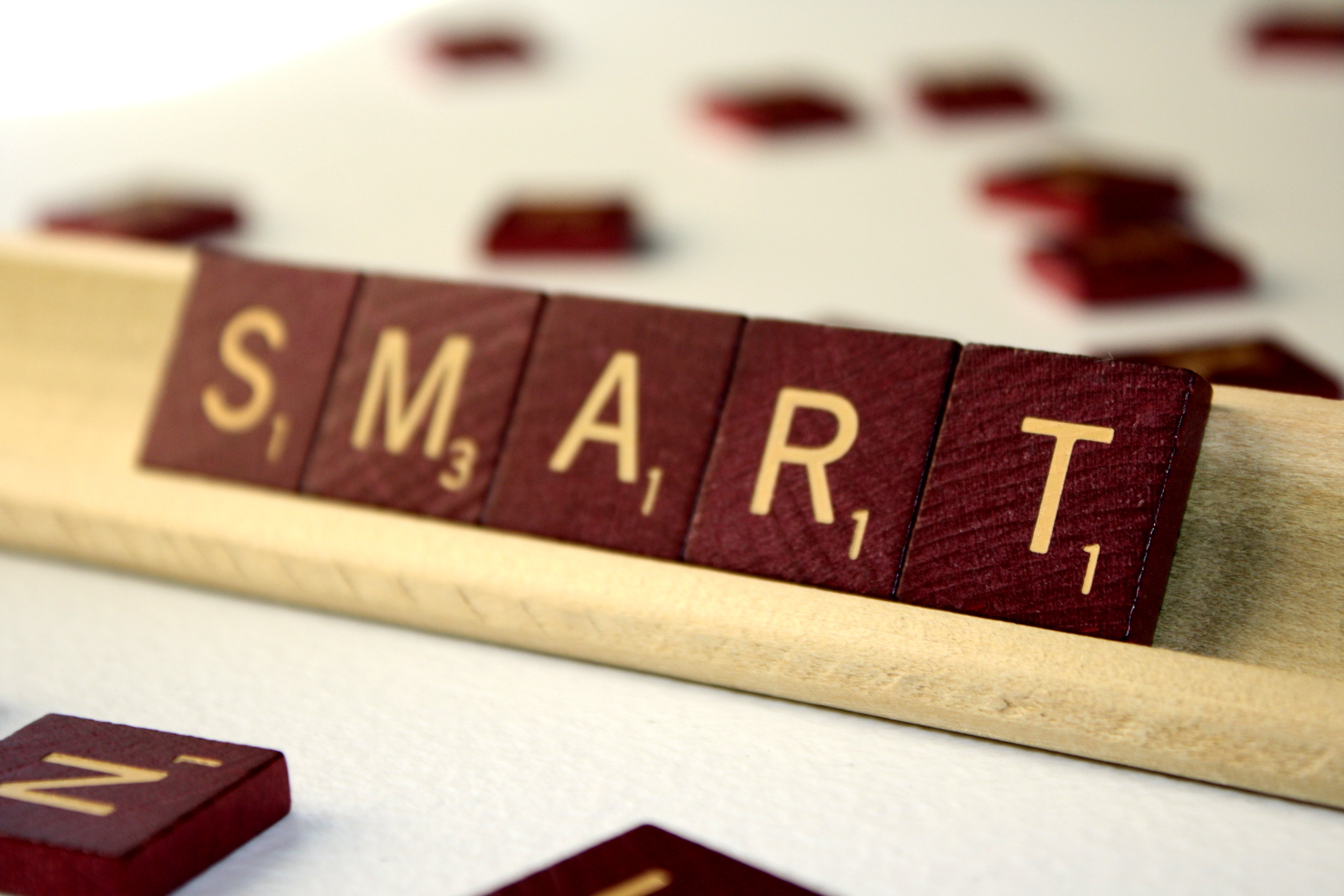
Retrieving Personal Data
By: Emergent2
April 26, 2023 12:43 AM / 0 Comments Education Self Evolution Philosophy
\
scssCopy code// Smart contract for retrieving personal data from the government
pragma solidity ^0.8.0;
contract PersonalDataRetrieval {
// Variables to store information about the person and the retrieval process
address payable public client;
address payable public personnel;
string public governmentAgency;
uint256 public retrievalFee;
bool public dataRetrieved;
// Events to track the retrieval process
event RetrievalInitiated(address client, address personnel, string governmentAgency, uint256 retrievalFee);
event RetrievalCompleted(address personnel);
event RetrievalCanceled(address client);
// Constructor to initialize the contract and set the retrieval fee
constructor(string memory _governmentAgency, uint256 _retrievalFee) payable {
client = payable(msg.sender);
governmentAgency = _governmentAgency;
retrievalFee = _retrievalFee;
dataRetrieved = false;
}
// Function to initiate the retrieval process and engage the personnel
function initiateRetrieval(address payable _personnel) public payable {
require(msg.sender == client, "Only the client can initiate the retrieval process");
require(!dataRetrieved, "Data has already been retrieved");
require(msg.value == retrievalFee, "The retrieval fee must be paid to initiate the process");
personnel = _personnel;
emit RetrievalInitiated(client, personnel, governmentAgency, retrievalFee);
}
// Function for the personnel to retrieve the data from the government agency
function retrieveData() public {
require(msg.sender == personnel, "Only the designated personnel can retrieve the data");
require(!dataRetrieved, "Data has already been retrieved");
// TODO: Call the appropriate function or API to retrieve the data from the government agency
dataRetrieved = true;
personnel.transfer(address(this).balance);
emit RetrievalCompleted(personnel);
}
// Function for the client to cancel the retrieval process and receive a refund
function cancelRetrieval() public {
require(msg.sender == client, "Only the client can cancel the retrieval process");
require(!dataRetrieved, "Data has already been retrieved");
client.transfer(address(this).balance);
emit RetrievalCanceled(client);
}
}
This smart contract includes the following features:
- The client can initiate the retrieval process by sending the specified retrieval fee to the contract and designating the personnel who will retrieve the data from the government agency.
- The personnel can retrieve the data by calling the
retrieveData()
function. - The client can cancel the retrieval process and receive a refund by calling the
cancelRetrieval()
function. - The contract includes events to track the retrieval process, including the initiation, completion, and cancellation of the process.
- The contract ensures that only the client can initiate the retrieval process and cancel it, and only the designated personnel can retrieve the data.
Note that this smart contract is a basic example and should be customized according to your specific needs and requirements. It is important to ensure that the retrieval process is legal and ethical, and that all parties involved comply with applicable laws and regulations.